開発環境
- macOS Mojave - Apple (OS)
- Emacs (Text Editor)
- Windows 10 Pro (OS)
- Visual Studio Code (Text Editor)
- Go (プログラミング言語)
プログラミング言語Go (ADDISON-WESLEY PROFESSIONAL COMPUTING SERIES) (Alan A.A. Donovan(著)、Brian W. Kernighan(著)、柴田 芳樹(翻訳)、丸善出版)の第1章(チュートリアル)、1.4(GIFアニメーション)、練習問題1.5の解答を求めてみる。
コード
package main import ( "fmt" "image" "image/color" "image/gif" "io" "math" "math/rand" "os" "time" ) var palette = []color.Color{color.RGBA{0x00, 0xFF, 0x00, 0xff}, color.Black} const ( whiteIndex = 0 blackIndex = 1 ) func main() { rand.Seed(time.Now().UTC().UnixNano()) for i := 0; i < 10; i++ { filename := fmt.Sprintf("sample5_%d.gif", i) file, err := os.Create(filename) if err != nil { fmt.Println(err) return } lissajous(file) file.Close() } } func lissajous(out io.Writer) { const ( cycles = 5 res = 0.001 size = 100 nframes = 64 delay = 8 ) freq := rand.Float64() * 3.0 anim := gif.GIF{LoopCount: nframes} phase := 0.0 for i := 0; i < nframes; i++ { rect := image.Rect(0, 0, 2*size+1, 2*size+1) img := image.NewPaletted(rect, palette) for t := 0.0; t < cycles*2*math.Pi; t += res { x := math.Sin(t) y := math.Sin(t*freq + phase) img.SetColorIndex(size+int(x*size+0.5), size+int(y*size+0.5), blackIndex) } phase += 0.1 anim.Delay = append(anim.Delay, delay) anim.Image = append(anim.Image, img) } gif.EncodeAll(out, &anim) }
入出力結果(Terminal, cmd(コマンドプロンプト))
$ go run sample5.go $
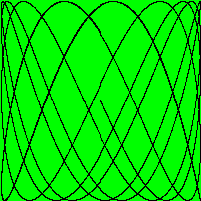
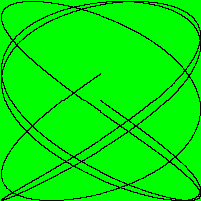
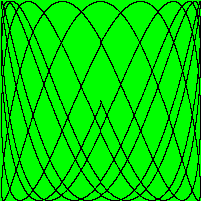
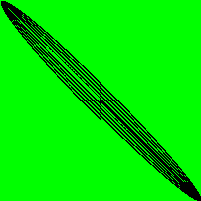
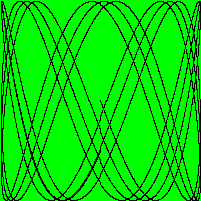
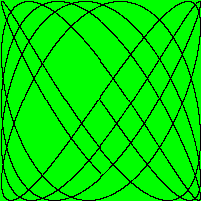
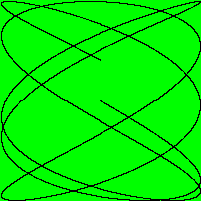
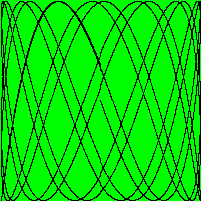
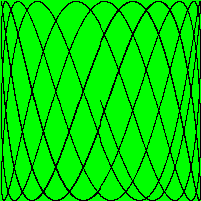
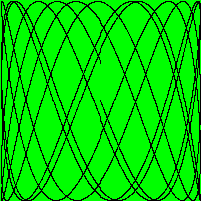
0 コメント:
コメントを投稿